FrozenSet<T> in .NET 8
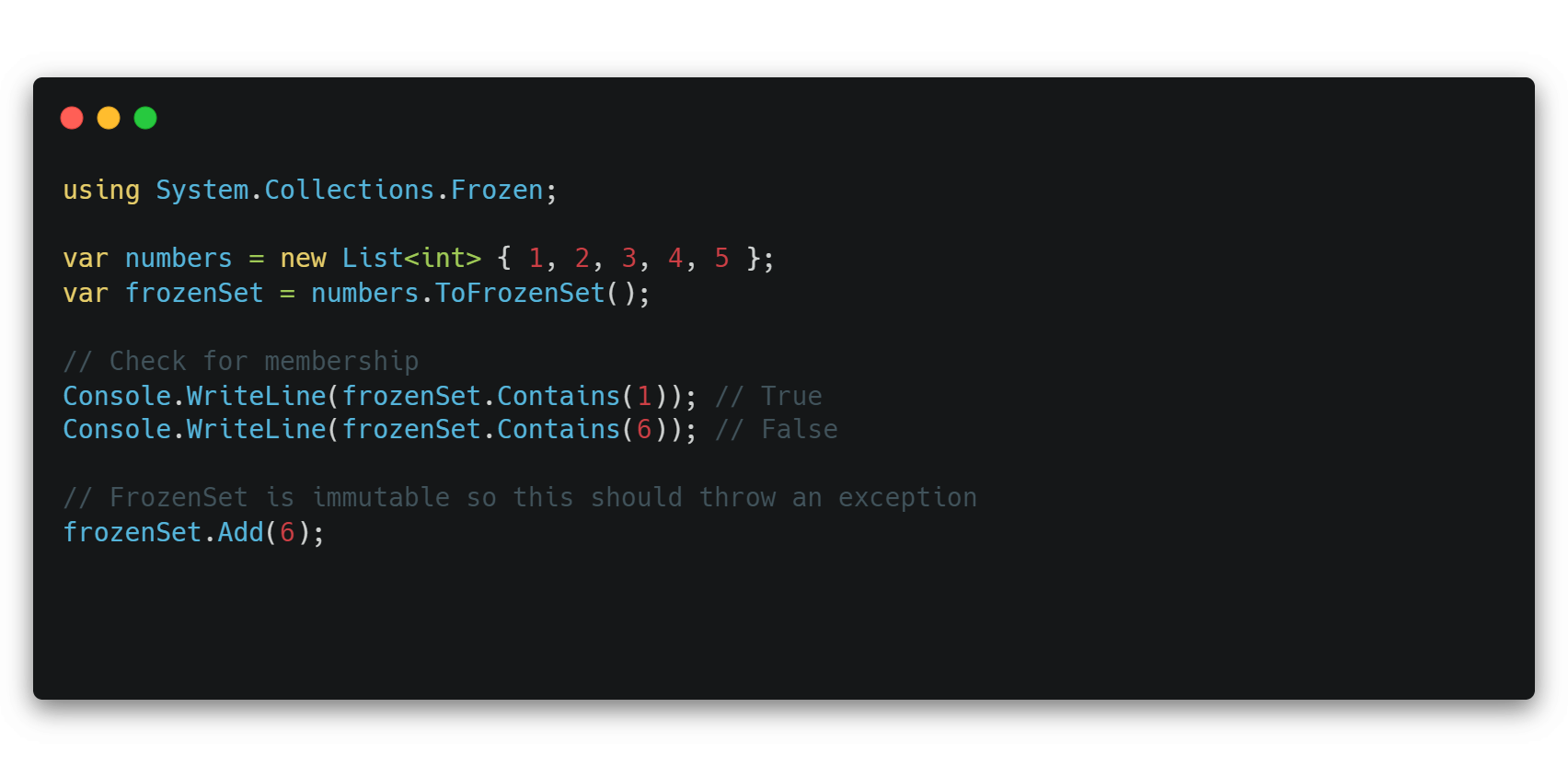
.NET 8 introduces a new type called FrozenSet<T>
, an immutable collection in the System.Collections.Frozen
namespace. Essentially, it represents a read-only set, meaning the elements within it cannot be added, removed, or modified after creation.
Key features:
Immutability: Once created, the content of the set remains unchangeable. This enhances thread safety and guards against unintended data corruption.
Lookup optimization: Thanks to its immutability, FrozenSet<T>
enhances performance for tasks such as checking membership and conducting intersection/union calculations.
Thread Safety: Its immutability makes FrozenSet<T>
inherently thread-safe, as multiple threads can access it without the risk of concurrent modifications.
Performance considerations and distinctions with other types
Generating a FrozenSet<T>
can be computationally more demanding when compared to mutable sets such as HashSet<T>
.
You should opt for FrozenSet<T>
when you need to create a set infrequently but access it frequently for lookups, especially in performance-critical scenarios.
What sets apart FrozenSet<T>
from ReadonlyCollection<T>
?
The primary distinction lies in the ability to modify the underlying collection. While it's feasible to alter the contents of a ReadOnlyCollection<T>
, such modifications are precluded in the case of FrozenSet<T>
. This fundamental dissimilarity underscores the importance of choosing the right immutable collection type based on the specific requirements of your application.
Use cases
One practical application is in the storage of configuration settings, particularly when these settings are loaded exclusively during the startup phase and experience minimal changes during runtime.
When managing a collection of configuration settings or constant values intended to remain immutable throughout the application's execution, leveraging FrozenSet<T>
ensures the preservation and integrity of these critical values. This proves especially beneficial in maintaining a stable and unalterable configuration state, aligning with best practices for configuration management.
Example
using System.Collections.Frozen;
var numbers = new List<int> { 1, 2, 3, 4, 5 };
var frozenSet = numbers.ToFrozenSet();
// Check for membership
Console.WriteLine(frozenSet.Contains(1)); // True
Console.WriteLine(frozenSet.Contains(6)); // False
// FrozenSet is immutable so this should throw an exception
frozenSet.Add(6);